What is __call__ in Python?
Python exposes a lot of built-in methods which can help us override almost any operation with the objects. One of those methods is __call__
method.
If we define a __call__
method then, it allows us to use the instance as a function.
class Demo:
def __init__(self):
print('instantiated a class')
def __call__(self):
print('instance is called')
a = Demo()
# instantiated a class
a()
# instance is called
In the above example, we can call the instance of Demo
class and then use it as a function call.
What are the advantages of using __call__
?
- A lot of libraries use
__call__
method for implementing a clear interface for APIs - Using
__call__
also, help you get the best of both worlds where you are using OOP advantage while using simple function call syntax. - There is a great StackOverflow answer mentioned below which highlights most of the use cases.
Python __call__ special method practical example
I know that __call__ method in a class is triggered when the instance of a class is called. However, I have no idea when I can use this special method, because one can simply create a new method and
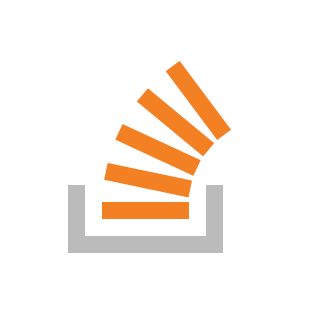