How to integrate Sentry for Django and Celery?
All developers want to write bug-free code and ship it to production. While developing on local we have stack trace to capture our errors and resolve them, but we don't have that privilege on a production server, then ultimately our end users will start experiencing bugs and we would not even know about it, until a client complaints about it. Now comes the most important question
How to capture errors on production? So that we get to know what errors our end users are facing and resolve it.
There are multiple tools in the market that can help you track and capture bugs and application failure in the production environment.
Out of all these, I have personally tried Sentry and prefer using Sentry for all my applications.
Let us see how we can integrate Sentry into our Django and Celery Application. To monitor and track all the bugs, exceptions on our production application
- Go to Sentry and signup

- Create a new project and select Django
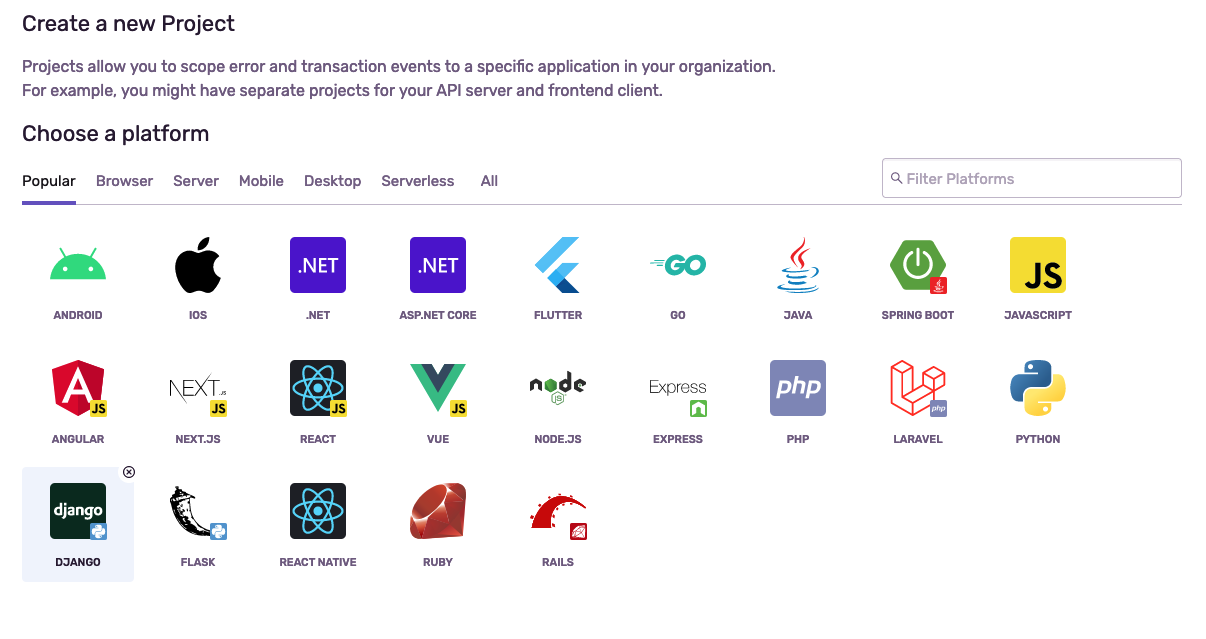
pip install sentry-sdk
install the sentry SDK in your project requirements, python virtual environment.- You would see a DNS link generated. Add the DNS link to your settings file with the following code
import sentry_sdk
from sentry_sdk.integrations.django import DjangoIntegration
sentry_sdk.init(
dsn="https://example@o123123.ingest.sentry.io/123123",
integrations=[DjangoIntegration()],
# If you wish to associate users to errors (assuming you are using
# django.contrib.auth) you may enable sending PII data.
send_default_pii=True
)
- Add the below URL to your main
urls.py
from django.urls import path
def trigger_error(request):
division_by_zero = 1 / 0
urlpatterns = [
path('sentry-debug/', trigger_error),
# ...
]
- Now hit the URL
https://<yourdomain>/sentry-debug
- Done!! You should be able to see your first error captured in the Sentry dashboard.
Since Celery also uses the same settings.py
file as Django uses, so Celery and Sentry integration would work seamlessly.
The above steps would help you do basic integration, but sentry does provide a lot of additional features which can help you track your code error better. Go to Sentry documentation to get an understanding of the details.
