Django Security middleware
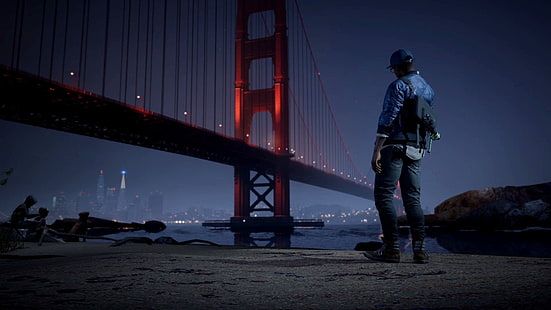
What is middleware?
Middleware is a program that acts as a bridge between two services/programs. Middleware should be like a plugin in any Framework.
Middleware in Django
Middleware is a framework of hooks into Django’s request/response processing.
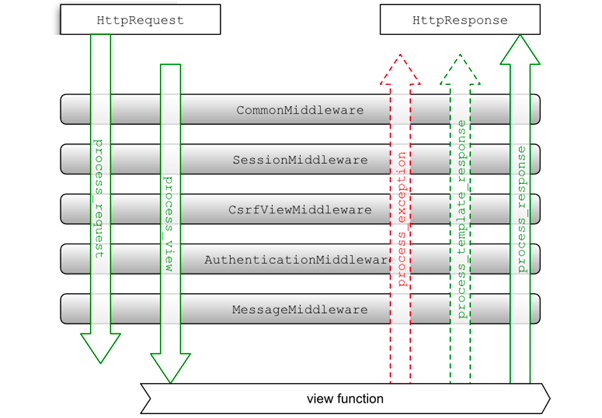
Django supports both Custom and Built-in Middleware.
This blog is limited to discussion on SecurityMiddleware
SecurityMiddleware is a class of Django Middleware
The django.middleware.security.SecurityMiddleware
provides several security enhancements to the request/response cycle.
The following list of settings can be set or updated in Django Settings
- SECURE_CONTENT_TYPE_NOSNIFF = True
- SECURE_CROSS_ORIGIN_OPENER_POLICY = "same-origin"
- SECURE_HSTS_INCLUDE_SUBDOMAINS = False
- SECURE_HSTS_PRELOAD = False
- SECURE_HSTS_SECONDS = 0
- SECURE_REDIRECT_EXEMPT = []
- SECURE_REFERRER_POLICY = "same-origin"
- SECURE_SSL_HOST = None
- SECURE_SSL_REDIRECT = False
Let's Discuss each of the settings in more detail.
SECURE_CONTENT_TYPE_NOSNIFF
The header X-Content-Type-Options is used in requests by a web server to protect you from MIME-type vulnerabilities. It implies only using provided content types and not guessing them.
- Default - True
- When set to True, it will set the header X-Content-Type-Options: nosniff.
- It should be set as many browsers try to assume the Content-type header and override the existing set value.
- This prevents the client from "sniffing" the asset to try and determine if the file type is something other than what is declared by the server.
SECURE_CROSS_ORIGIN_OPENER_POLICY
This setting sets the header Cross-Origin-Opener-Policy
. This option will help you to determine what access the top-level document has over the cross-origin document. when this option is set, documents do not share the same environment as cross-origin documents.
- Default - "same-origin"
Allowed Options
same-origin
- Isolates the browsing context exclusively to same-origin documents. Cross-origin documents are not loaded in the same browsing context. This is the default and most secure option.
same-origin-allow-popups
- Isolates the browsing context to same-origin documents or those which either don’t set COOP or which opt out of isolation by setting a COOP of unsafe-none.
unsafe-none
- Allows the document to be added to its opener’s browsing context group unless the opener itself has a COOP of same-origin or same-origin-allow-popups.
SECURE_HSTS_SECONDS
This setting sets the header Strict-Transport-Security
. HTTP Strict Transport Security (HSTS) is set to tell web browsers
- that communication should always be over TLS/SSL, so it transfers links from http to https
- In case of any certificate validation issues, the connection should be terminated.
- Default - 0 i.e do not set
Strict-Transport-Security
header - To enable it, we have to set it to a non-integer value
- The recommended time is 1 year to ensure security for max duration
- Syntax: Strict-Transport-Security: max-age=<expiry-time>;
Note: The first request is not protected, if first session is hijack, attacker can successfully conduct attack on initial connection
SECURE_HSTS_INCLUDE_SUBDOMAINS
This setting appends includeSubDomains
to Strict-Transport-Security
header. It enforces HSTS Policy(explained above) to all the subdomains
- Default - False
- To enable it, it should be set to True
- Example: Strict-Transport-Security: max-age=; includeSubDomains
SECURE_HSTS_PRELOAD
Setting max-age in the HSTS header does not make it 100% secure as the first request is unprotected. HSTS provides a setting option of preload where you can submit your domain to https://hstspreload.org/ which adds your domain to preload list managed by Google.
Once approved, your domain will have an HSTS header even before visiting for the first time.
- Default - False i,e no preload option is set
- If set to true, It will update the header Strict-Transport-Security by adding the parameter preload
- Example: Strict-Transport-Security: max-age=; includeSubDomains; preload
- When using preload, the max-age directive must be at least 31536000 (1 year), and the includeSubDomains directive must be present.
SECURE_REDIRECT_EXEMPT
- Default - []
- Prerequisite - SECURE_SSL_REDIRECT should be True
- If a URL path matches a regular expression in this list, the request will not be redirected to HTTPS. The SecurityMiddleware strips leading slashes from URL paths, so patterns shouldn’t include them, e.g. SECURE_REDIRECT_EXEMPT = [r'^no-ssl/$', …]
SECURE_REFERRER_POLICY
- Default - "same-origin"
- It will set header Referrer-Policy
Allowed Values
no-referrer
- Instructs the browser to send no referrer for links clicked on this site.
no-referrer-when-downgrade
- Instructs the browser to send a full URL as the referrer, but only when no protocol downgrade occurs.
origin
- Instructs the browser to send only the origin, not the full URL, as the referrer.
origin-when-cross-origin
- Instructs the browser to send the full URL as the referrer for same-origin links, and only the origin for cross-origin links.
same-origin
- Instructs the browser to send a full URL, but only for same-origin links. No referrer will be sent for cross-origin links.
strict-origin
- Instructs the browser to send only the origin, not the full URL, and to send no referrer when a protocol downgrade occurs.
strict-origin-when-cross-origin
- Instructs the browser to send the full URL when the link is same-origin and no protocol downgrade occurs; send only the origin when the link is cross-origin and no protocol downgrade occurs; and no referrer when a protocol downgrade occurs.
unsafe-url
- Instructs the browser to always send the full URL as the referrer.
SECURE_SSL_HOST
- Default - None
- Prerequisite - SECURE_SSL_REDIRECT should be True
- If value is set, all the requests will be forwarded to this host other than host present in original request.
SECURE_SSL_REDIRECT
- Default - False
- If this is set to true, all the non-HTTP requests will be transferred to HTTPS requests.
- Exception: It will bypass URLs present in SECURE_REDIRECT_EXEMPT.